AFD-200 - Flutter
Application
Development
Overview
Flutter is an open-source user interface software development kit (app SDK) created by Google. It is for building high
performance, high-fidelity apps for iOS Android, and web from a single codebase. The purpose of this course if to enable
developers create high-performance and attractive apps that feel natural on iOS and Android devices. Flutter is used by
companies around the world including Alibaba, Capital One, and Groupon for apps that touch hundreds of millions of users.
Course Objective
- Be highly productive:
• Develop for iOS and Android from a single codebase.
• Do more with less code, even on a single OS, with a modern, expressive language, and a declarative approach.
• Prototype and iterate easily where you can change your code and reload it as your app runs (hot reload feature)
as you will see in the next lessons. Also, Flutter fixes crashes and continue debugging from where the app is left
off. - Create beautiful, highly customized user experiences (UI):
• Benefit from a rich set of Material Design and Cupertino (iOS-flavour) widgets built using Flutter’s own framework.
• Realize custom, beautiful, brand-driven designs, without the limitations of OEM widget sets.
Who Should Attend
Prerequisites
Before attending this course, students must have:
- A basic understanding of programming concepts and logic (e.g., variables, loops, conditionals, and functions).
- Familiarity with object-oriented programming (OOP) principles is recommended, but not mandatory.
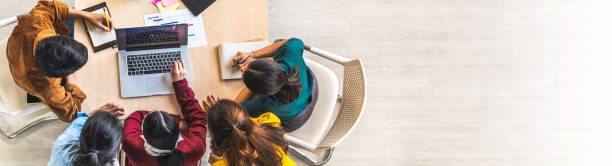
Training Calendar
Intake
Duration
Program Fees
Module
Module 1 - Introduction to Flutter and Dart Programming Language
- Introduction
- Â Importance of Flutter
- Â Introduction to Dart
- Â Writing Dart code
- Â DartPad
- Â Installing Dart SDK
- IntelliJ IDEA
- Lab 1: Installing Dart IDEA and Writing Dart ProgramÂ
– Installing IntelliJ IDEA
– Crating a Dart Project Using IntelliK IDEAÂ
– Using DartPad
Module 2 - Dart Programming – Syntax
- Introduction
- Main () function
- Dart Variables
- Dart Data Types
- Input of Information to Dart Program
- Writing Comments
- Dart Conditional Operators
- If Statement
- If – Else Statement
- If…Else and Else…If… Statement
- If Else and Logical Operators
- For Loops
- While Loops
- Do-while Loops
- Break Statement
- Switch Case Statement
- Lab 2 Create a Pizza Order Program
Module 3 - Dart Functions & Object-Oriented Programming (OOP)
• Functions
– Function Structure
– Creating a Function
-Function Return Data Types
-Void Function
-Function Returning Expression
-Functions and Variables Scope
• Object-Oriented Programming (OOP)
-ObjectÂ
-Class
-Creating a Class
-Adding Methods to Classes
-Class Inheritance
-Class – Getters and Setters
-Abstract Class
• Dart Project Structure and Dart Libraries
• Lab 3: Create a Small overtime Payment Program
Module 4 - Introduction to Flutter
- Understanding Flutter
- Flutter Framework
- Android Studio
- What is Android Studio?
- Android Studio Software Prerequisite
- Installing Android Studio
- Flutter SDK
- Installing and Configuring Flutter SDK
- Creating a New Flutter Project
- Setup and Android Virtual Device
- Run a Flutter App
- Installing Flutter on Mac
- Test Your Flutter App on iOS Phone with Windows O.S
- Android Studio Sugar and Spice
- Run your Apps on a Hardware Device (Physical Phone)
- Run your Flutter App on Android Phone
- Run your Flutter App on iPhone Device
- Emulator Debug Mode
- Introduction to Flutter Widgets
- Creating a Flutter App Using Widgets
- What is a MaterialApp widget?
- Lab 4: Creating a Simple Flutter App
Module 5 - Flutter Widgets Fundamentals
- Scaffold Widget
- Image Widget
- Container Widget
- Column and Row Widgets
- Con Widget
- Layouts in Flutter
- Card Widget
- App Icons for iOS and Android Apps
- Hot Reload and Hot Restart
- Stateful and Stateless Widgets
- Use a Custom Font
- Lab 5: Creating a Restaurant Menu
Module 6 - Navigation and Routing
- Button Widget
- FloatingActionButton
- RaisedButton, FlatButton, and IconButton
- DropdownButton
- OutlineButton
- ButtonBar
- PopupMenuButton
- App Structure and Navigation
- Navigate to a New Screen and Back
- Navigate with Named Routes
- Send and Return Data Among Screens
- Animate a Widget Across Screens
- WebView Widget in Flutter
- Lab 6: Navigation and Routing a Pizza Store App
Module 7 - Visual, Behavioural, and Motion-Rich Widgets Implementing Material Design Guidelines – Part 1
- Introduction
- BottomNavigatorBar Widget
- DefaultTabController, TabBar and TabBarView Widgets
- ListTile Widget
- ListView Widget
- Drawer Widget
- DataTable Widget
- SelectableText Widget
- Stack Widget
- Lab A: Creating a Flutter App Using BottomNavigatorBar Navigation Technique
- Lab B: Using DataTable Sorting Built-In Function
Module 8 - Visual, Behavioural, and Motion-Rich Widgets Implementing Material Design Guidelines – Part 2
- Input and Selections
-Text Field Widget
-CheckboxGroup and
-RadioButtonGroup Widgets
-Date Picker
-Time Picker
-Slider Widget
-Switch Widget - Dialogs, Alerts, and Panels
-Alert Dialog Widget
-Cupertino Alert Dialog Widget
-Bottom Sheet
-Modal Bottom Sheet
-Persistent Bottom Sheet
-Expansion Panel Widget
-Snack Bar Widget - Â Lab 8: Creating a Hotel Reservation App
Module 9 - Firebase
- Introduction
- What is the JSON?
- How does Firebase Database work?
- Firebase authentication (Signup and Login to Flutter App)
- Configure Your App to use Firebase Services-
Adding Firebase to your Android App
-Adding Firebase to your iOS App - Configuring Firebase Authentication
-Login to an App Using Firebase User Accounts
-Logout Configuration - Firebase Database
–Â Â Which database is right for your project?
-Real Time Database
-Cloud Firestore - Lab 9: Create a User Profile Interface using Firebase
Module 10 - Location-Aware Apps: Using GPS and Google Maps
- Introduction
- What is the JSON?
- How does Firebase Database work?
- Firebase authentication (Signup and Login to Flutter App)
- Configure Your App to use Firebase Services
- Adding Firebase to your Android App
- Adding Firebase to your iOS App
- Configuring Firebase Authentication
- Login to an App Using Firebase User Accounts
- Logout Configuration
- Firebase Database
- Which database is right for your project?
- Real Time Database
- Cloud Firestore
- Lab 9: Create a User Profile Interface using Firebase
FAQs
General Questions:
- Q: What is Flutter?
A: Flutter is an open-source UI toolkit developed by Google for building natively compiled applications for mobile, web, and desktop from a single codebase. It uses the Dart programming language and provides a rich set of pre-designed widgets for creating beautiful, high-performance apps. - Q: What is this course about?
A: This course teaches you how to effectively use Flutter and Dart to develop cross-platform mobile applications for both Android and iOS. - Q: Who is this course for?
A: This course is designed for individuals who are new to mobile app development or developers looking to build cross-platform applications using a single codebase. It is suitable for software developers, web developers, and anyone interested in creating high-performance mobile apps with Flutter. - Q: What are the prerequisites for this course?
A: Basic programming knowledge is recommended, but not required. Familiarity with object-oriented programming concepts will be helpful. - Q: What software do I need?
A: You’ll need Flutter SDK, Dart SDK, and an integrated development environment (IDE) like Android Studio or Visual Studio Code. - Q: How is the course structured?
A: The course may include in-person workshops, live online sessions, self-paced online modules, or a combination of these formats. Check the course details for specifics. - Q: How long is the course?
A: The duration varies depending on the format and depth of content. It can range from a few days to several weeks. Check the course details for exact duration. - Q: Will I receive a certificate upon completion?
A: Yes.
Program Content & Skills:
Q: What specific topics are covered in the course?
A: Typical topics include setting up Flutter, understanding the Flutter framework, building user interfaces with widgets, managing state, handling navigation, working with APIs and databases, and deploying applications. Check the syllabus for a detailed list.
Q: Will I learn about advanced features of Flutter?
A: The course primarily focuses on core functionalities and practical applications for building cross-platform apps. Advanced topics like animations, custom widgets, and performance optimization might be covered in separate, advanced courses.
Q: Will I learn how to integrate Flutter with backend services?
A: Yes, the course will cover integrating Flutter with APIs, Firebase, and other backend services to build dynamic and data-driven applications.
Q: Will I work on real-world examples and exercises?
A: Yes, the course includes hands-on exercises, projects, and real-world examples to help you apply the concepts and build functional applications.
Submit your interest today !