JavaScript Advanced
Programming and Ajax
Overview
Course Objective
Upon successful completion of this course, students will be able to:
• Create and manage custom classes.
• Control program flow by writing code to respond to specific criteria.
• Implement object-oriented programming techniques to create reusable and reliable programs.
• Work with Java utility class libraries.
• Use the capabilities of the Java I/O package to read and write data to external files or media.
• Use collection APIs in Java to manage data.
• Use generic to enforce compile-time type checking.
• Use multi-threaded programs for memory efficiency and create distributable versions of a Java application.
Who Should Attend
Prerequisites
• Java Fundamentals (Java SE 7)
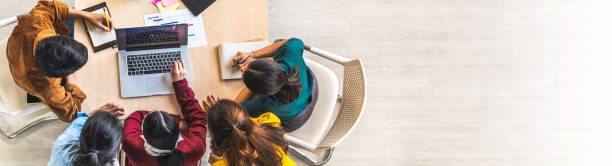
Training Calendar
Intake
Duration
Program Fees
Module
Module 1 - Using Programming Techniques
• Topic 1A: Maintain JavaScript Code
• Topic 1B: Debug JavaScript Code
Module 2 - Implementing Cross-Browser Compatibility
• Topic 2A: Provide Support for Multiple Browsers
• Topic 2B: Enable JavaScript
• Topic 2C: Detect Browser and JavaScript Versions
• Topic 2D: Detect Objects
• Topic 2E: Handle Exceptions
Module 3 - Using Custom Objects in JavaScript
• Topic 3A: Create Custom Objects
• Topic 3B: Use Constructor Functions
• Topic 3C: Add Methods to Objects
• Topic 3D: Use Prototype Functions
Module 4 - Working with Arrays
• Topic 4A: Create Arrays
• Topic 4B: Delete Array Elements
• Topic 4C: Use Multidimensional Arrays
Module 5 - Handling Cookies Using JavaScript
• Topic 5A: Use Cookie Objects
• Topic 5B: Store Cookies
• Topic 5C: Process Cookie Values
• Topic 5D: Delete Cookies
Module 6 - Validating Forms Using JavaScript
• Topic 6A: Validate Forms Using the Generic Approach
• Topic 6B: Implement Generic Validation Using Custom Objects
Module 7 - Programming Using DOM API
• Topic 7A: Traverse Documents
• Topic 7B: Modify Documents
• Topic 7C: Append Nodes to Documents
Module 8 - Communicating with Plus-Ins and Server Applications
• Topic 8A: Communicate with Adobe Flash Content
• Topic 8B: Communicate with ActiveX
• Topic 8C: Communicate with Server Applications
Module 9 - Using Ajax
• Topic 9A: Create and XMLHttpRequest Object
• Topic 9B: Fetch Information from Servers using the XML
HttpRequest Object
Module 10 - Creating Applications with Ajax
• Topic 10A: Crate an Auto-Complete Application Using Ajax
• Topic 10B: Interact with a Database Using Ajax
• Topic 10C: Validate Forms Using Ajax
• Topic 10D: Work with Third-Party Ajax Libraries
FAQs
General Questions:
Q: What is the JavaScript Advanced Programming and Ajax course about?
This 2-day course is designed for experienced web developers who want to enhance their skills in JavaScript programming and Ajax. You will learn advanced JavaScript techniques such as creating and managing custom objects, working with arrays, handling cookies, validating forms, and implementing cross-browser compatibility. The course also focuses on using Ajax for dynamic web applications, including creating auto-complete features, interacting with databases, and using third-party Ajax libraries to improve performance and interactivity on web pages.
Q: Who should attend this course?
This course is intended for experienced web developers who are already familiar with XHTML, basic JavaScript, and web browsers. It is perfect for those looking to take their JavaScript skills to the next level by learning advanced techniques and implementing Ajax to create dynamic, interactive web applications.
Q: What are the prerequisites?
Participants should have experience with JavaScript programming, be comfortable creating web pages, and proficient in using web browsers such as Internet Explorer. It is recommended that participants complete the JavaScript Programming (Fourth Edition) course or have equivalent knowledge.
Q: How long is the course?
The course is 2 full days.
Q: What will I learn in this course?
In this course, you will learn to:
Maintain and debug JavaScript code
Implement cross-browser compatibility and handle exceptions
Create and manage custom objects, including using constructor functions and prototype methods
Work with arrays, including multidimensional arrays and array element deletion
Handle cookies in JavaScript (storing, processing, and deleting cookies)
Validate forms using generic and custom approaches
Program with the DOM API to traverse and modify web documents
Communicate with external content like Adobe Flash, ActiveX, and server applications
Use Ajax to fetch information from servers and build interactive applications
Create an auto-complete feature, interact with databases, and validate forms using Ajax
Q: Will I get a certificate after the course?
Yes. Upon successful completion of the course, you will receive a Certificate of Completion.
Program Content & Skills:
Q: What skills will I gain from the JavaScript Advanced Programming and Ajax course?
You will gain advanced JavaScript programming skills, including creating and managing custom objects, working with arrays, and handling cookies. You’ll also learn how to implement cross-browser compatibility, handle exceptions, and work with the DOM API to dynamically manipulate web pages. In addition, you’ll gain expertise in using Ajax for server communication, such as fetching data, creating auto-complete features, and interacting with databases, enabling you to build highly interactive and dynamic web applications.
Q: Will I learn how to write JavaScript programs in this course?
Yes. This course will guide you through writing complex JavaScript programs, starting with custom object creation and progressing to interactive applications using Ajax. You’ll work on improving program flow, handling errors, and implementing reusable code using object-oriented principles. By the end of the course, you’ll be able to design, write, and debug advanced JavaScript applications.
Q: Can I learn how to implement object-oriented principles in this course?
Yes. The course covers key object-oriented concepts in JavaScript, such as creating custom objects, using constructor functions, and adding methods through prototypes. You’ll also learn to manage program complexity using reusable and reliable code with object-oriented programming principles.
Q: Is error handling covered in the course?
Yes. This course covers error handling in JavaScript, including managing exceptions, detecting browser issues, and writing code that handles runtime errors. You’ll learn how to make your JavaScript applications more resilient and reliable through proper error handling techniques.
Q: Will I be able to apply JavaScript programming skills in real-world scenarios after the course?
Absolutely. This course focuses on practical, real-world applications of advanced JavaScript programming. By the end of the course, you’ll have the skills to create dynamic, interactive web applications, manage server-side communication with Ajax, and optimize performance across multiple browsers. These skills will be applicable to a wide range of web development projects.
Submit your interest today !